HttpClient ODPS connection based on json response like success how to integrate with ThomsonReute...
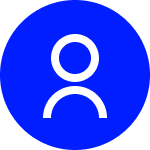
...rs RFA
How to connect HttpClient class of opendac like below class to RFA API class
public class HttpClient
{
public static void Main(string[] args)
{
string url = "";
while (true)
{
string curLine = "";
string urlError = "Please enter a valid URL";
Console.Write("Enter ODPS URL > ");
try
{
curLine = Console.ReadLine().Trim();
}
catch (IOException)
{
Environment.Exit(0);
}
if (curLine.Length < 8 || !curLine.StartsWith("http://"))
{
Console.WriteLine(urlError);
continue;
}
if (!curLine.EndsWith("/"))
{
url = curLine + "/";
}
else
{
url = curLine;
}
break;
}
bool usePersistent = true;
while (true)
{
string curLine = "";
string inputError = "Please enter only a character from y, Y, n, and N";
Console.Write("Use persistent connection (y, n) > ");
try
{
curLine = Console.ReadLine().Trim();
}
catch (IOException)
{
Environment.Exit(0);
}
if (curLine.Equals("n", StringComparison.OrdinalIgnoreCase))
{
usePersistent = false;
}
else if (!curLine.Equals("y", StringComparison.OrdinalIgnoreCase))
{
Console.WriteLine(inputError);
continue;
}
break;
}
if (!usePersistent)
{
ServicePointManager.Expect100Continue = false;
ServicePointManager.DefaultConnectionLimit = 1000; // You can adjust this value based on your requirement
ServicePointManager.MaxServicePoints = 1000; // You can adjust this value based on your requirement
ServicePointManager.UseNagleAlgorithm = false;
ServicePointManager.EnableDnsRoundRobin = true;
ServicePointManager.SetTcpKeepAlive(false, 0, 0);
}
while (true)
{
Console.Write("ODPS command > ");
string curLine = Console.ReadLine().Trim();
if (curLine.Length == 0)
{
continue;
}
if (curLine.StartsWith("q", StringComparison.OrdinalIgnoreCase))
{
break;
}
string sendUrl = url + curLine;
try
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(sendUrl);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
using (Stream dataStream = response.GetResponseStream())
{
StreamReader reader = new StreamReader(dataStream);
string responseFromServer = reader.ReadToEnd();
Console.WriteLine(responseFromServer);
}
response.Close();
}
catch (WebException e)
{
if (e.Status == WebExceptionStatus.ProtocolError)
{
Console.WriteLine("Error contacting ODPS: " + ((HttpWebResponse)e.Response).StatusCode);
}
else
{
Console.WriteLine("Error contacting ODPS: " + e.Message);
}
}
catch (Exception e)
{
Console.WriteLine("Error contacting ODPS: " + e.Message);
}
}
}
}
Best Answer
-
Hi @rakeshgowdacs,
You can use the OpenDACS API instead of ODPS to integrate with RFA. Depending on your use case, much of the user DACS entitlement checking can also be done automatically by the RFA/RTMDS. Please see this article to learn more about the use of OpenDACS API which is build on top of RFA SDK package.
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 690 Datastream
- 1.4K DSS
- 629 Eikon COM
- 5.2K Eikon Data APIs
- 11 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 255 ETA
- 560 WebSocket API
- 39 FX Venues
- 15 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 25 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 280 Open PermID
- 45 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 23 RDMS
- 2K Refinitiv Data Platform
- 721 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 106 UPA
- 194 TREP Infrastructure
- 229 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 95 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛