Hi there,
I am currently testing the Eikon API for the first time so I do not have much experience with it yet. I put together a little python function for testing, which is meant to give me data for a list of stocks and hence returns identifiers, names, and some fundamental data. The code I used for this is attached below, which currently uses the constituent of the SXXP Index to pull some data (individual app key needs to be provided)...
Now when running this file I bump into 2 problems sometimes:
1.) Eikon Request gives me error, even for small
requests
- Sometimes
the code runs into an error out of nowhere (sometimes it works just
fine). I get the following error message, which is basically an error code 400,
“Bad Request Error” coming from the “json_requestl.py” file and the function
“check_server_error”. - Why do those server errors occur? I initially thought (and by reading through some other posts here) I thought the request was too large in one go, but even changing to the DAX universe with 30 names bumps into this problem occasionaly...
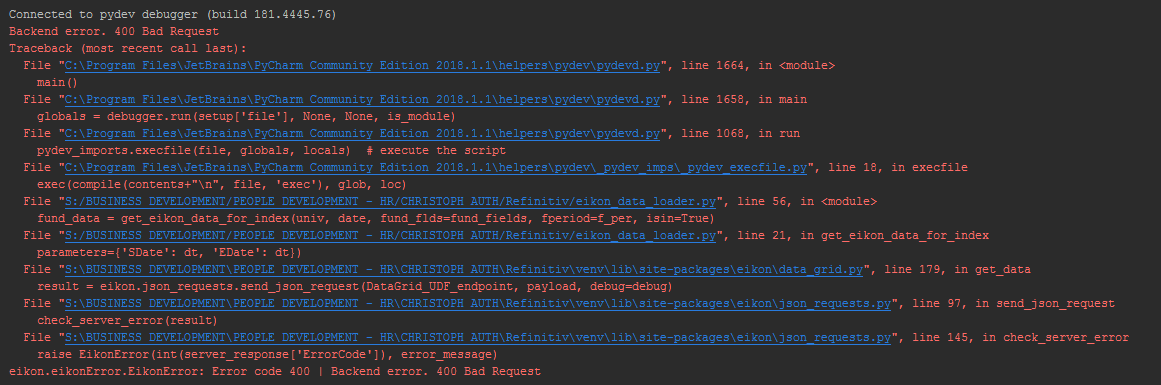
2.) Eikon gives me
weird behavior when changing input fields
- The following function (which is also comes from the code below) let's me pull RIC's and Names for the constituents of the SXXP as of Jan 2017:
inst_list, _ =
ek.get_data(instruments=['.STOXX'],
fields=['TR.IndexConstituentRIC', 'TR.IndexConstituentName'],
parameters={'SDate': '2017-01-15', 'EDate': '2017-01-15'})
- when continuing a statement after this, I can pull other data using the obtained RIC's, where I can use TR.ISINCode or TR.Sedol to get two different further identifiers (just as an example here). When running the fields individually, I get a complete list of identifiers for each constituent.
isin_list, _ =
ek.get_data(instruments=inst_list.loc[:,'Constituent
RIC'], fields=['TR.ISINCode'])
- this is all cool so far, however, the problem comes when I try to pull ISIN and Sedol at the same time. Again, this doesnt happen all the time and just occaionally, I get an incomplete list of the two identifiers like the one below, where ISINs and/or SEDOLs are missing sometimes, where I would get some when I run this individually for each field.
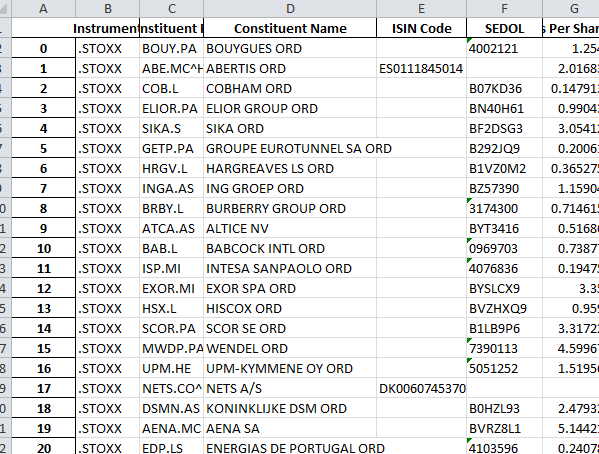
So far, me pulling data feels quite instable. Once I bump into these problems, the only thing that seems to resolve this is me closing and re-opening the Python IDE. (I'm on Pycharm btw.)
Would you be able to help on this/would you know what I am doing wrong?
Many thanks.
Best,
Chris
import eikon as ek
def get_eikon_data_for_index(universe, dt, eikon_key=None, ric_codes=None, fund_flds=None, fperiod=None, isin=False, xl_name=None):
if sum(x is None for x in [fund_flds, fperiod]) == 1:
ValueError('Fundamental fields and list must both be defined!')
if eikon_key is None:
eikon_key = 'Need to set own key here...'
ek.set_app_key(eikon_key)
if ric_codes is None:
static_flds = ['TR.IndexConstituentRIC', 'TR.IndexConstituentName']
inst_list, err_const = ek.get_data(instruments=universe, fields=static_flds,
parameters={'SDate': dt, 'EDate': dt})
else:
inst_list = ric_codes
# get the list of stocks within the STOXX 600 universe...
if isin:
isin_list, err_isin = ek.get_data(instruments=inst_list.loc[:, 'Constituent RIC'].tolist(), fields=['TR.ISINCode', 'TR.SEDOL'])
inst_list[isin_list.columns[1:]] = isin_list.iloc[:, 1:]
if fperiod is not None:
fund_data, err_fund = ek.get_data(instruments=inst_list.loc[:, 'Constituent RIC'].tolist(), fields=fund_flds, parameters={'SDate': dt, 'Period': fperiod})
else:
fund_data, err_fund = ek.get_data(instruments=inst_list.loc[:, 'Constituent RIC'].tolist(), fields=fund_flds, parameters={'SDate': dt})
inst_list[fund_data.columns[1:]] = fund_data.iloc[:, 1:]
# write this over into a spreadsheet
if xl_name is not None:
inst_list.to_excel(xl_name)
return inst_list
if __name__== '__main__':
date = '2017-01-15'
univ = ['.STOXX']
# get some fundamentals here...
fund_fields = ['TR.EPSMean', 'TR.OperatingMarginPercent']
f_per = 'FY0'
fund_data = get_eikon_data_for_index(univ, date, fund_flds=fund_fields, fperiod=f_per, isin=True)
# get dividend yield data...
div_yld = ['TR.DPSMeanYield']
f_per = 'FY1'
div_yld = get_eikon_data_for_index(univ, date, ric_codes=fund_data.loc[:, ['Constituent RIC']], fund_flds=div_yld, fperiod=f_per, isin=False)
# get Net Debt/EBITDA data...
n_d_to_ebitda = ['TR.NetDebtToEBITDA']
net_debt_to_ebitda = get_eikon_data_for_index(univ, date, ric_codes=fund_data.loc[:, ['Constituent RIC']],fund_flds=n_d_to_ebitda, isin=False)
#combine data...
fund_data[div_yld.columns[1:]] = div_yld.iloc[:, 1:]
fund_data[net_debt_to_ebitda.columns[1:]] = net_debt_to_ebitda.iloc[:, 1:]
# write to excel...
fund_data.to_excel('test_point-in-time.xlsx')