DACS RFA OpenAPI AuthorizationSystem Does not fall back to next connection in List
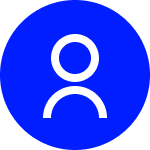
I am setting up fallback logic for a DACS Open API system using a comma separated list for the "dacs.daemon" AuthorizationSystem property.
When there is no network connection to the first item in the list, this works as expected and makes a connection to the second item. However when the first item in the list returns a connection down, as we have hit the 8 connection limit on that server it does not fall back to the second item.
My code:
public DACSClient(String daemonHostRed, String daemonHostBlue)
{
this.daemonHost = daemonHostRed + "," + daemonHostBlue;
try
{
openDACSConnection();
getDACSAgent();
}
catch (AuthorizationException e)
{
LOGGER.error("Unable to acquire DACS Authorization system", e);
//Without the DACS we can do nothing.
onAuthorizationSystemNotAvailableEvent();
}
}
private void openDACSConnection() throws AuthorizationException
{
if (dacsEventQueue == null)
{
dacsEventQueue = EventQueue.create("dacsEventQueue");
executorService = Executors.newSingleThreadScheduledExecutor(dacsFactory);
executorService.scheduleAtFixedRate(this::dispatchEventQueue, 0, 100, TimeUnit.MICROSECONDS);
}
if (dacsSystem != null)
{
//If the system is already up do nothing
return;
}
AuthorizationSystem.setProperty("dacs.daemon", daemonHost);
dacsSystem = AuthorizationSystem.acquire();
}
private void getDACSAgent() throws AuthorizationException
{
dacsAgent = dacsSystem.createAuthorizationAgent("DACS Entitlements Service", true);
try
{
while (dacsAgent.daemonConnectionState() == AuthorizationConnection.CONNECTION_PENDING)
{
//noinspection BusyWait
Thread.sleep(500);
}
if (dacsAgent.daemonConnectionState() == AuthorizationConnection.CONNECTION_UP)
{
//No way exposed in the dacs openapi to get which of the red/blue we are actually connected to.
LOGGER.info("DACS Connection UP");
getDACSService();
return;
}
}
catch (InterruptedException e)
{
LOGGER.error(e.getMessage(), e);
throw new AuthorizationException(e.getMessage());
}
LOGGER.error("DACS Connection DOWN");
closeConnection(true);
throw new AuthorizationException("DACS Connection DOWN");
}
We are NOT using the AuthorizationMCSystem, where I would expect to drop the CONNECTION_DOWN item from the list of available connections, and I do not want to make multiple connections.
What am I missing here?
Answers
-
Thank you for reaching out to us.
As far as I know, this is an expected behavior.
The flow should look like this:
The OpenDACS API could establish a connection to the first DACS daemon and the DACS daemon accepted the connection. Then the DACS daemon cut the connection due to its connection limit. In this case, the OpenDACS API will keep reconnect to the first DACS daemon because the first DACS daemon is running and it can accept incoming connections.
If you are a named user of the Refinitiv Developer Connect (API support), you can contact the API support team direclty via Contact Premium Support to confirm this behavior.
0 -
Thanks Jirapongse
In that case I will implement the MC variant of the auth system to move between connections.
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 684 Datastream
- 1.4K DSS
- 615 Eikon COM
- 5.2K Eikon Data APIs
- 10 Electronic Trading
- Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 249 ETA
- 554 WebSocket API
- 37 FX Venues
- 14 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 23 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 275 Open PermID
- 44 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 22 RDMS
- 1.9K Refinitiv Data Platform
- 643 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 26 DACS Station
- 121 Open DACS
- 1.1K RFA
- 104 UPA
- 192 TREP Infrastructure
- 228 TRKD
- 915 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 90 Workspace SDK
- 11 Element Framework
- 5 Grid
- 18 World-Check Data File
- 1 Yield Book Analytics
- 46 中文论坛