[RDP Authentication Inquiry] refresh_token Not Working Properly
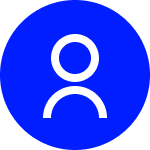
Hi, I am using Python to issue and use an RDP Authentication Token.
However, during my testing, I found that when I send a request with grant_type='refresh_token'
, the same access_token
and refresh_token
are returned in the response as those sent in the request. As a result, even when I send a request with grant_type='refresh_token'
, the tokens expire after 5 minutes.
Below is the code from my testing. I attempted refreshing every minute, but as you can see from the logs, the same access_token
and refresh_token
are continuously returned. Eventually, after 5 minutes—or sometimes even earlier—I receive a 400 error. Could this be due to a contractual limitation or restriction on the use of the refresh_token
?
refresh_interval = 60 auth_url = "https://api.refinitiv.com:443/auth/oauth2/v1/token" data = { 'username': username, 'password': password, 'grant_type': 'password', 'takeExclusiveSignOnControl': 'true', 'scope': 'trapi'} response = requests.post(auth_url, headers={'Accept': 'application/json'}, data=data, auth=(client_id, ''), verify=True, allow_redirects=False) response.raise_for_status() auth_response = response.json() print("[create_token] Refinitiv Data Platform Authentication succeeded. RECEIVED:") print(json.dumps(auth_response, sort_keys=True, indent=2, separators=(',', ':'))) refinitiv_token_info = { "access_token": auth_response["access_token"], "refresh_token": auth_response["refresh_token"], "expired_time": time.time() + refresh_interval } print(f"[create_token] Token created successfully.") print(json.dumps(refinitiv_token_info, sort_keys=True, indent=2, separators=(',', ':'))) token_for_refresh = refinitiv_token_info["refresh_token"] while 1: data = {'refresh_token': token_for_refresh, 'grant_type': 'refresh_token'} print("[refresh_token] Sending authentication request with refresh token to", auth_url, "...") print(f"[refresh_token] post data: {data}") response = requests.post(auth_url, headers={'Accept': 'application/json'}, data=data, auth=(client_id, '') ) response.raise_for_status() auth_response = response.json() print("[refresh_token] Refinitiv Data Platform Authentication succeeded. RECEIVED:") print(json.dumps(data, sort_keys=True, indent=2, separators=(',', ':'))) refinitiv_token_info = { "access_token": auth_response['access_token'], "refresh_token": auth_response['refresh_token'], "expired_time": time.time() + refresh_interval } print(f"[refresh_token] Token created successfully.") print(json.dumps(refinitiv_token_info, sort_keys=True, indent=2, separators=(',', ':'))) token_for_refresh = refinitiv_token_info["refresh_token"] print('-'*150) time.sleep(60)
Answers
-
Thank you for reaching out to us.
I ran the code and found that the refresh token will be the same but the access tokens are different.
The first part of access tokens are quite similar. You need to check the last part. For example:
print(auth_response["access_token"][-10:])
You may need to add the login to compare the access tokens in the code.
0 -
Hello @younaDev,
I am not sure if you want to use streaming service (RTO), or just REST API, but there are working Python and Postman samples which can get you started quickly and overcome these trivial issues.
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 37 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 698 Datastream
- 1.5K DSS
- 633 Eikon COM
- 5.2K Eikon Data APIs
- 14 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 6 Trading API
- 2.9K Elektron
- 1.5K EMA
- 257 ETA
- 565 WebSocket API
- 40 FX Venues
- 16 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 25 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 283 Open PermID
- 47 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 24 RDMS
- 2.1K Refinitiv Data Platform
- 803 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 122 Open DACS
- 1.1K RFA
- 107 UPA
- 194 TREP Infrastructure
- 232 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 100 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛