Downloading data by industry code
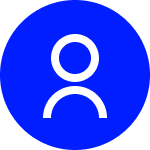
Hello,
I was sent here after opening a ticket at the help desk.
I am trying to download data through python for a certain number of industries for a few specific variables. Here is the code that I'm using but it's not working.
import eikon as ek
import pandas as pd
# Set your Eikon API key
ek.set_app_key('4c19fc66a2524a0f990085ea12a37f58816bce51')
# List of industry codes (based on your previous request)
industry_codes = [
'5510','1510', '4020', '2520', '1510', '5520', '1510', '1010', '4510', '1010',
'2010', '2530', '2010', '4010', '4020', '2030', '3020', '101020', '2030', '4520',
'101020', '2010', '4520', '2520', '3010', '6010', '2020', '2510', '151040', '3020',
'2530', '2030', '3020', '2530', '4020', '2550', '2520', '4030', '5020', '3520',
'5530', '4520', '2550', '2520', '4030', '5020', '2530', '1510'
]
# List of variables to retrieve for each industry
variables = [
'TR.CompanyName', 'TR.TRESGEmissionsScore', 'TR.PolicyEmissions', 'TR.AnalyticCO2',
'TR.AnalyticTotalWaste', 'TR.ClimateChangeRisksOpp', 'TR.AnalyticHazardousWaste',
'TR.AnalyticWasteRecyclingRatio', 'TR.AnalyticCO2IndirectScope3', 'TR.EnvPartnerships',
'TR.TargetsEmissions', 'TR.EnvRestorationInitiatives'
]
# Initialize an empty DataFrame to store all the data
all_data = pd.DataFrame()
# Loop through all industry codes and retrieve the data
for industry_code in industry_codes:
try:
# Query data for the current industry code
data = ek.get_data(f'I:{industry_code}', variables)
# Add a column for the industry code for tracking
data['IndustryCode'] = industry_code
# Append the data to the all_data DataFrame
all_data = pd.concat([all_data, data], ignore_index=True)
# Print progress (optional)
print(f"Data for industry {industry_code} retrieved successfully.")
except Exception as e:
print(f"Error retrieving data for industry {industry_code}: {e}")
# Save the data to a CSV file (optional)
all_data.to_csv('industry_data.csv', index=False)
# Display a snippet of the data
print(all_data.head())
I'm getting this error:
Error retrieving data for industry 5510: 'tuple' object does not support item assignment
2025-04-03 13:54:35,311 P[93699] [MainThread 8020811904] Backend error. 400 Bad Request2025-04-03 13:54:35,313 P[93699] [MainThread 8020811904] HTTP request failed: EikonError-Backend error. 400 Bad Request
Error retrieving data for industry 1510: Error code 400 | Backend error. 400 Bad Request
2025-04-03 13:54:54,510 P[93699] [MainThread 8020811904] UDF Core request failed. Gateway Time-out2025-04-03 13:54:54,512 P[93699] [MainThread 8020811904] HTTP request failed: EikonError-UDF Core request failed. Gateway Time-out
Error retrieving data for industry 4020: Error code 2504 | UDF Core request failed. Gateway Time-outError retrieving data for industry 2520: 'tuple' object does not support item assignmentError retrieving data for industry 1510: 'tuple' object does not support item assignment
2025-04-03 13:59:41,445 P[93699] [MainThread 8020811904] Backend error. 400 Bad Request2025-04-03 13:59:41,447 P[93699] [MainThread 8020811904] HTTP request failed: EikonError-Backend error. 400 Bad Request
Error retrieving data for industry 5520: Error code 400 | Backend error. 400 Bad Request
......
I'm not sure what I'm doing wrong.
This is what the help desk said:
Hello Nicholas,
I appreciate the additional details you shared.
We have reviewed the syntax/ formula you have shared and the error message together with our Product Specialist team and we have identified that this is a script error which need to be raised in Developer portal. Developer portal will have experts in API that can assist.
Thank you,
Nicholas
Answers
-
Hi @Nicholas_D ,
May I ask what instruments the I's stand for? for e.g.: I:5510?
I'm asking becasue I cannot find them in the DIB. If these are not valid RICs, they cannot nessesarily be used in calling data via our Python libraries.
Also, I would advise using the updated version of the library; the LDL, since the Eikon Data API in your example will be deprecated this year.
0 -
I thought these were the industry codes, I could be wrong:
industry_codes = [
'5510','1510', '4020', '2520', '1510', '5520', '1510', '1010', '4510', '1010',
'2010', '2530', '2010', '4010', '4020', '2030', '3020', '101020', '2030', '4520',
'101020', '2010', '4520', '2520', '3010', '6010', '2020', '2510', '151040', '3020',
'2530', '2030', '3020', '2530', '4020', '2550', '2520', '4030', '5020', '3520',
'5530', '4520', '2550', '2520', '4030', '5020', '2530', '1510'
]
I'm trying to download data by industry, I never have before, so I think I'm doing it wrong and I'm not sure how to fix the problem. Basically, I'm trying to get data for organized by industry for several years for the variables identified above and I'm running into some issues.Thank you for your quick response.
0 -
Hi @Nicholas_D ,
Please note that this LSEG Developer Q&A Forum is for LSEG API technical questions only. For content questions, such as ones looking for the RICs representing Industry Codes, please use myaccount.lseg.com.
With that said, I think you may Screeners… Is that what you're after?
https://github.com/LSEG-API-Samples/Example.DataLibrary.Python/blob/lseg-data-examples/Tutorials/1.Access/LD%20Lib.%20Python%20Tutorial%209%20-%20Screeners.ipynb
0 -
They are the ones that sent me here.
0 -
The get_data method doesn't work with the industry_codes. It requires instrument codes, such as RICs, CUSIPs, ISINs, etc.
Therefore, you need to use the Screener or Search API to search for instrument codes, such RICs. Then, use RICs with the get_data method to retrieve data.
I am not sure what those industry codes are:
Are they TRBC or NAICS?
0 -
Thank you very much!
Actually, I think I found the wrong list of industry codes, but this is getting closer to what I'm trying to do.
I want to download data by industry, not by firm , for the variables listed above. For example all the firms for a particular Industry Group. My understanding is category scores are organized by Industry Group. So, I'm trying to download data by Industry group to compare category scores but I'm struggling to get the info organized in such a way.
Sorry, maybe I'm not asking my question very clearly.
For example, I'm trying to find out how I could download the data for these variables for these industry groups for a specific year or range of years in python:
variables = [
'TR.CompanyName', 'TR.TRESGEmissionsScore', 'TR.PolicyEmissions', 'TR.AnalyticCO2',
'TR.AnalyticTotalWaste', 'TR.ClimateChangeRisksOpp', 'TR.AnalyticHazardousWaste',
'TR.AnalyticWasteRecyclingRatio', 'TR.AnalyticCO2IndirectScope3', 'TR.EnvPartnerships',
'TR.TargetsEmissions', 'TR.EnvRestorationInitiatives'
]Industry groups[
Natural Gas Utilities
Office Equipment
Oil & Gas
Oil & Gas Related Equipment and Services
Paper & Forest Products
]
0 -
Hi @Nicholas_D
Yes, in this case what you're after is the Screener:
https://github.com/LSEG-API-Samples/Example.DataLibrary.Python/blob/lseg-data-examples/Tutorials/1.Access/LD%20Lib.%20Python%20Tutorial%209%20-%20Screeners.ipynb
An exampe use would be:
import lseg.data as ld ld.open_session() from dataquery import *
screen = SCREEN.express.universe(
Equity(active=True, public=True, primary=True)).conditions(
IN('TR.NAICSIndustryGroupAllCode', "5415"),
IN('TR.CoRTradingCountryCode', 'US'),
TOP(field='TR.CompanyMarketCap', bound=10, metric=nnumber)
).currency('USD').query
df = ld.get_data(
universe=screen,
fields=['TR.CompanyName', 'TR.TRESGEmissionsScore', 'TR.PolicyEmissions', 'TR.AnalyticCO2',
'TR.AnalyticTotalWaste', 'TR.ClimateChangeRisksOpp', 'TR.AnalyticHazardousWaste',
'TR.AnalyticWasteRecyclingRatio', 'TR.AnalyticCO2IndirectScope3', 'TR.EnvPartnerships',
'TR.TargetsEmissions', 'TR.EnvRestorationInitiatives'])
display(df)screen here is:
'SCREEN(U(IN(Equity(primary,public,active))),IN(TR.NAICSIndustryGroupAllCode,5415),IN(TR.CoRTradingCountryCode,US),TOP(TR.CompanyMarketCap,10,nnumber),CURN=USD)'
For more information on the screen used above, please look into:https://github.com/LSEG-API-Samples/Example.DataLibrary.Python/blob/lseg-data-examples/Tutorials/1.Access/LD%20Lib.%20Python%20Tutorial%209%20-%20Screeners.ipynb
1
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 685 Datastream
- 1.4K DSS
- 615 Eikon COM
- 5.2K Eikon Data APIs
- 10 Electronic Trading
- Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 252 ETA
- 556 WebSocket API
- 38 FX Venues
- 14 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 23 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 275 Open PermID
- 44 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 22 RDMS
- 1.9K Refinitiv Data Platform
- 650 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 104 UPA
- 193 TREP Infrastructure
- 228 TRKD
- 917 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 90 Workspace SDK
- 11 Element Framework
- 5 Grid
- 18 World-Check Data File
- 1 Yield Book Analytics
- 46 中文论坛