Why do I have values for get_data() and not for get_timeseries?
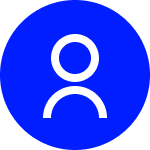
For get_data I have values for TLSA.O for the fields I have chosen.
#import packages
import eikon as ek # the Eikon Python wrapper package
import pandas as pd
import numpy as np
import datetime
from datetime import timedelta, date, datetime
from dateutil.relativedelta import relativedelta
#connects to Bill's Eikon terminal
ek.set_app_key('72d2821f21064a0b8890860db39e375eacd87e24')
df = pd.DataFrame(df)
df = ek.get_data('TSLA.O',
['TR.NumOfStrongBuy',
'TR.NumOfBuy',
'TR.NumOfHold',
'TR.NumOfSell',
'TR.NumOfStrongSell'])
print(df)
Output:
( Instrument Recommendation - Number Of Strong Buy \ 0 TSLA.O 6 Recommendation - Number Of Buy Recommendation - Number Of Hold \ 0 3 11 Recommendation - Number Of Sell Recommendation - Number Of Strong Sell 0 10 3 , None)
However, when requesting this data using get_timeseries all of the outputs are NaN. Why is this and how do I get it to give numbers in the same way that get_data() does?
#get dates and format as strings
end_date = datetime.now()
start_date = end_date - relativedelta(months=1)
end_date_str = datetime.strftime(end_date, "%Y-%m-%d")
start_date_str = datetime.strftime(start_date, "%Y-%m-%d")
#retreive time series
df = pd.DataFrame()
df = ek.get_timeseries('TSLA.O',
['TR.NumOfStrongBuy',
'TR.NumOfBuy',
'TR.NumOfHold',
'TR.NumOfSell',
'TR.NumOfStrongSell'],
start_date = start_date_str,
end_date = end_date_str)
df
Output:
TSLA.OTR.NUMOFSTRONGBUYTR.NUMOFBUYTR.NUMOFHOLDTR.NUMOFSELLTR.NUMOFSTRONGSELLDate2020-06-12NaNNaNNaNNaNNaN2020-06-15NaNNaNNaNNaNNaN2020-06-16NaNNaNNaNNaNNaN2020-06-17NaNNaNNaNNaNNaN2020-06-18NaNNaNNaNNaNNaN2020-06-19NaNNaNNaNNaNNaN2020-06-22NaNNaNNaNNaNNaN2020-06-23NaNNaNNaNNaNNaN2020-06-24NaNNaNNaNNaNNaN2020-06-25NaNNaNNaNNaNNaN2020-06-26NaNNaNNaNNaNNaN2020-06-29NaNNaNNaNNaNNaN2020-06-30NaNNaNNaNNaNNaN2020-07-01NaNNaNNaNNaNNaN2020-07-02NaNNaNNaNNaNNaN2020-07-06NaNNaNNaNNaNNaN2020-07-07NaNNaNNaNNaNNaN2020-07-08NaNNaNNaNNaNNaN2020-07-09NaNNaNNaNNaNNaN2020-07-10NaNNaNNaNNaNNaN
Best Answer
-
Hello @bill39
get_timeseries(..) does not support the fields you specified above. The function supports only 'TIMESTAMP', 'VALUE', 'VOLUME', 'HIGH', 'LOW', 'OPEN', 'CLOSE', 'COUNT'. This is explained in Eikon Data APIs for Python - Reference Guide . That's why you get NaN.
You can specify start date and end date in get_data(..) if the fields supports date. You can check if a field supports date or not using "Data Item Browser" tool. Please refer to the answer of this question which shows how to use the tool.
I have checked your preference fields using "Data Item Browser" and the fields support date. The snipped example source code shows how to set start date and end date for the fields:
end_date = datetime.now()
start_date = end_date - relativedelta(months=1)
end_date_str = datetime.strftime(end_date, "%Y-%m-%d")
start_date_str = datetime.strftime(start_date, "%Y-%m-%d")
print("start="+start_date_str + " ,end="+end_date_str)
#return dataframe and errors
test_1,err= ek.get_data('TSLA.O',
['TR.NumOfStrongBuy.date', #show date
'TR.NumOfStrongBuy',
'TR.NumOfBuy',
'TR.NumOfHold',
'TR.NumOfSell',
'TR.NumOfStrongSell'],
{'SDate':start_date_str, 'EDate':end_date_str}) #set start and end date
#show data
test_1The example output:
0
Answers
-
Hello @bill39
The document is correct. get_timeseries(..) has the parameters start_date and end_date but it provides only 'TIMESTAMP', 'VALUE', 'VOLUME', 'HIGH', 'LOW', 'OPEN', 'CLOSE', 'COUNT' field as an example shown in Eikon Data API Quick Start guide below:
If you would like to get other fields apart from get_timeseries(..) provides, you need to use get_data(..)
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 690 Datastream
- 1.5K DSS
- 629 Eikon COM
- 5.2K Eikon Data APIs
- 11 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 255 ETA
- 560 WebSocket API
- 39 FX Venues
- 15 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 25 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 281 Open PermID
- 46 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 23 RDMS
- 2K Refinitiv Data Platform
- 723 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 106 UPA
- 194 TREP Infrastructure
- 229 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 95 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛