Here's some sample working code, just have to set your variable for the Eikon API key:
from typing import Union, List
import pandas as pd
import eikon as ek
import os
os.environ['HTTP_PROXY'] = ''
os.environ['HTTPS_PROXY'] = ''
def get_bid_ask(id_list: Union[List[str], str], start_date: str, end_date: str, api_key: str) -> pd.DataFrame:
ek.set_app_key(api_key)
if isinstance(id_list, str):
id_list = [id_list]
fields = ['TR.BIDPRICE', 'TR.ASKPRICE', 'TR.ASKPRICE.calcdate', 'TR.FiCurrency']
parameters = {'Frq': 'D', 'Sdate': start_date, 'Edate': end_date}
df, _ = ek.get_data(instruments = id_list, fields = fields, parameters = parameters)
# Broadcast currency since currency is only returned on first date
df['Currency'] = df.groupby('Instrument')['Currency'].transform('first')
# Drop rows with NA
df = df.dropna()
# Filter for dates - there is a bug where more dates than those inputted are returned.
# Example: id_list = 'XS2078642183', start_date = '2022-07-22', end_date = '2022-07-22'
df = df.loc[ (df['Calc Date'] >= start_date) & (df['Calc Date'] <= end_date) ]
return df
id_list = "XS1713193586"
asofdate = "2022-10-05"
test = get_bid_ask(id_list, start_date=asofdate, end_date=asofdate, api_key=api_key)
Here's the output:
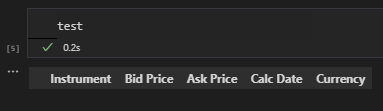
Here's the available Bid/Ask price in Excel:
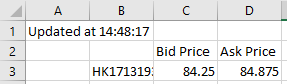
Using the formula:
=@TR("HK171319358=","TR.BIDPRICE; TR.ASKPRICE","SDate=2022-10-05 CH=Fd RH=IN",B2)
Might be a mapping issue, please advice.
Many thanks.