Refinitiv.data proper fields names
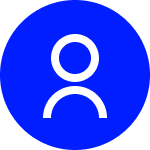
Greetings,
I am currently involved in a project aimed at retrieving the closing price for companies that have been delisted due to bankruptcy, specifically the day following their bankruptcy announcement. The primary methodology I employ involves checking whether 'TR.MnAHasBankruptcy' is true. If this condition is met, we proceed to use 'TR.MnAHasBankruptcy.date' to extract the closing price on the subsequent day, utilizing "TR.PriceCloseDate".
I have encountered a challenge in executing the code intended for this purpose. For reference, the dataframe columns available for extraction are as follows: Index(['Instrument', 'Delisted Quote Flag', 'RetireDate', 'Bankruptcy Flag', 'Date', 'Acquisition Techniques', 'Date Effective or Unconditional', 'Price Close', 'Date'], dtype='object'). It is noteworthy that both 'TR.MnAHasBankruptcy.date' and "TR.PriceCloseDate" correspond to the same field name "Date".
I am seeking a solution to address this ambiguity between field names. What strategies could potentially resolve this issue?
Thank you!
import refinitiv.data as rd
import pandas as pd
rd.open_session()
results = rd.discovery.search(
view=rd.discovery.Views.EQUITY_QUOTES,
top=500,
filter="(IssuerOAPermID eq '4295899979' and RCSAssetCategoryLeaf eq 'Ordinary Share')",
select="RIC, DTSubjectName, RCSAssetCategoryLeaf, ExchangeName, ExchangeCountry"
)
df = results
substrings = ['.OQ', '.O', '.N', '.A']
def is_valid_ric(ric):
for ending in substrings:
if ending in ric:
pos = ric.find(ending)
if len(ric) == pos + len(ending) or (len(ric) > pos + len(ending) and ric[pos + len(ending)] == '^'):
return True
return False
filtered_df = df[df['RIC'].apply(is_valid_ric)]
rics = filtered_df['RIC'].tolist()
df = rd.get_data(
universe=rics,
fields=[
'TR.IsDelistedQuote',
'DELIST_DAT',
'TR.RetireDate',
'TR.MnAHasBankruptcy',
'TR.MnAHasBankruptcy.date',
"TR.MnAAcquisitionTechnique(Concat='|')",
'TR.MnADateUnconditional',
"TR.PriceClose",
"TR.PriceCloseDate",
],
parameters={}
)
# Filtering DataFrame where 'TR.MnAHasBankruptcy' is True
bankruptcy_df = df[df['Bankruptcy Flag'] == True].copy()
# Adding a new column for the target date, which is one day after 'TR.MnAHasBankruptcy.date'
bankruptcy_df['TargetDate'] = pd.to_datetime(bankruptcy_df['TR.MnAHasBankruptcy.date']) + pd.Timedelta(days=1)
# Prepare a DataFrame to hold the final results
final_columns = ['RIC', 'TR.MnAHasBankruptcy.date', 'TargetDate', 'Price Close']
final_df = pd.DataFrame(columns=final_columns)
# Iterate through the bankruptcy DataFrame
for index, row in bankruptcy_df.iterrows():
target_date = row['TargetDate']
ric = row['RIC']
# Attempt to find the 'TR.PriceClose' where 'TR.PriceCloseDate' matches 'TargetDate'
price_close_row = df[(df['RIC'] == ric) & (pd.to_datetime(df['TR.PriceCloseDate']) == target_date)]
if not price_close_row.empty:
price_close = price_close_row.iloc[0]['Price Close']
final_df = final_df.append({
'RIC': ric,
'TR.MnAHasBankruptcy.date': row['TR.MnAHasBankruptcy.date'],
'TargetDate': target_date,
'Price Close': price_close
}, ignore_index=True)
else:
# Handle the case where no matching 'TR.PriceClose' is found
final_df = final_df.append({
'RIC': ric,
'TR.MnAHasBankruptcy.date': row['TR.MnAHasBankruptcy.date'],
'TargetDate': target_date,
'Price Close': "<NA>"
}, ignore_index=True)
print(final_df)
final_df.to_csv("./bankruptcy_price_close.csv")
rd.close_session()
Best Answer
-
Hi @vitali ,
If I understand your request properly, you would simply just like to diferenciate between the two 'Date' columns? Do let me know if I missunderstood.
There are 2 ways that come to mind. The 1st is to use the `use_field_names_in_headers` argument:df = rd.get_data(
universe=rics,
fields=[
'TR.IsDelistedQuote',
'DELIST_DAT',
'TR.RetireDate',
'TR.MnAHasBankruptcy',
'TR.MnAHasBankruptcy.date',
"TR.MnAAcquisitionTechnique(Concat='|')",
'TR.MnADateUnconditional',
"TR.PriceClose",
"TR.PriceCloseDate",
],
parameters={},
use_field_names_in_headers=True)The 2nd is to rename the columns with
for i in range(len(my_list)):
if my_list[i] == 'Date':
my_list[i] = replacement_values[count]
count += 1
if count == len(replacement_values):
breakIt's rather ugly but it does the trick:
import refinitiv.data as rd
import pandas as pd
rd.open_session()
results = rd.discovery.search(
view=rd.discovery.Views.EQUITY_QUOTES,
top=500,
filter="(IssuerOAPermID eq '4295899979' and RCSAssetCategoryLeaf eq 'Ordinary Share')",
select="RIC, DTSubjectName, RCSAssetCategoryLeaf, ExchangeName, ExchangeCountry"
)
df = results
substrings = ['.OQ', '.O', '.N', '.A']
def is_valid_ric(ric):
for ending in substrings:
if ending in ric:
pos = ric.find(ending)
if len(ric) == pos + len(ending) or (len(ric) > pos + len(ending) and ric[pos + len(ending)] == '^'):
return True
return False
filtered_df = df[df['RIC'].apply(is_valid_ric)]
rics = filtered_df['RIC'].tolist()
df = rd.get_data(
universe=rics,
fields=[
'TR.IsDelistedQuote',
'DELIST_DAT',
'TR.RetireDate',
'TR.MnAHasBankruptcy',
'TR.MnAHasBankruptcy.date',
"TR.MnAAcquisitionTechnique(Concat='|')",
'TR.MnADateUnconditional',
"TR.PriceClose",
"TR.PriceCloseDate",
],
parameters={},)
my_list = df.columns.to_list()
replacement_values = ["MnAHasBankruptcyDate", "PriceCloseDate"]
count = 0
for i in range(len(my_list)):
if my_list[i] == 'Date':
my_list[i] = replacement_values[count]
count += 1
if count == len(replacement_values):
break
df.columns = my_list
df0
Answers
-
Greetings, @jonathan.legrand!
Thank you for your prompt response.Upon reviewing your feedback, I've realized that it doesn't fully address my requirements. To provide some context, my code utilizes specific field names like 'Bankruptcy Flag' and 'Price Close', which are accurately retrieved using the "df.columns" method. However, I've retained the original names 'TR.MnAHasBankruptcy.date' and 'TR.PriceCloseDate' unchanged because both include the term 'Date', leading to potential conflicts. Due to this similarity, crafting an algorithm that distinguishes between these similarly named fields poses a challenge, as it results in errors.
So, if I leave 'TR.MnAHasBankruptcy.date' and 'TR.PriceCloseDate' unchanged I receive an error:If I replace 'TR.MnAHasBankruptcy.date' and 'TR.PriceCloseDate' with their proper name 'Date', the error is:
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 689 Datastream
- 1.4K DSS
- 626 Eikon COM
- 5.2K Eikon Data APIs
- 11 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 255 ETA
- 558 WebSocket API
- 39 FX Venues
- 15 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 23 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 277 Open PermID
- 44 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 23 RDMS
- 2K Refinitiv Data Platform
- 705 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 106 UPA
- 194 TREP Infrastructure
- 229 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 94 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛