get_data does not return quarterly data for some fields
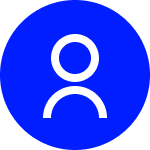
Good evening,
I have a problem using the Eikon API on Python.
I wrote a function that extracts different fields for a given company and that organizes them according to the dates in a dataframe. I want to retrieve quarterly data. The problem is that for some fields the function get_data returns correctly the data for each quarter (december, march, june, september), while for other fields (e.g., TR.Revenue) it returns the data only for the month of december of each year. I checked the fundamentals of the company through Refinitiv Workspace and the data that I want to extract are available from there for each quarter. Can somebody help me? This is the code:
def extract_data(company_tickers, fields_to_extract, custom_fields_option, standard_options, print_info=False, save=False):
df_list = []
while len(custom_fields_option) < len(fields_to_extract):
custom_fields_option.append({})
for i, field in enumerate(fields_to_extract):
extracted_field, error = ek.get_data(company_tickers, [field+'.date', field if bool(custom_fields_option[i]) == False else custom_fields_option[i]], standard_options)
extracted_field = extracted_field.drop('Instrument', axis=1)
for row in range(len(extracted_field)):
extracted_field['Date'].iloc[row] = extracted_field['Date'].iloc[row][:7]
extracted_field = extracted_field.set_index('Date')
extracted_field = extracted_field.drop_duplicates()
df_list.append(extracted_field)
if error:
print("ERRORS:", error)
if print_info:
print("Extracted data at iteration:",i)
print(extracted_field)
concatenated_df = pd.DataFrame()
for i, df in enumerate(df_list):
if i == 0:
concatenated_df = df
else:
concatenated_df = concatenated_df.join(df, how='left')
concatenated_df = concatenated_df.rename(columns={"Net Income Incl Extra Before Distributions": "Net Income", "Price Target - Mean": "Price Target",
"Total Shareholders' Equity incl Minority Intr & Hybrid Debt": "Tot Shareholders Equity",
"Net Cash - Ending Balance": "Net Cash"})
if print_info:
print(concatenated_df)
if concatenated_df.index[0] > concatenated_df.index[1]:
if print_info:
print("FLIP APPLIED")
concatenated_df = concatenated_df.iloc[::-1].copy()
concatenated_df = concatenated_df.fillna(method='ffill')
concatenated_df = concatenated_df.dropna(axis=0)
concatenated_df = concatenated_df.astype(float)
if save:
concatenated_df.to_csv('DATA.csv', header=True, index=True)
return concatenated_df
The function is called in this way:
company_ticker = 'AZMT.MI'
field_to_extract = ['TR.PriceClose', 'TR.Revenue', 'TR.GrossProfit', 'TR.NetIncome', 'TR.RevenueMedian', 'TR.PriceTargetMean', 'TR.F.TOTASSETS', 'TR.F.TOTLIAB', 'TR.F.TOTSHHOLDEQ', 'TR.F.NETCASHENDBAL']
custom_field_option = [{'TR.PriceClose' : {'params':{'Scale': 0}}}]
standard_option = {'Scale': 6, 'SDate': 0, 'EDate': -130, 'FRQ': 'Q', 'Curn': 'EUR'}
df1 = extract_data(company_ticker, field_to_extract, custom_field_option, standard_option, True, False)
While the function extracts the data it prints the retreived data, here you can see for example that the field 'TR.PriceClose' works correctly giving results for all the different quarters.
Unfortunately, the most of the fields behave like 'TR.Revenue', giving me only the value for the month of december.
Best Answer
-
Problem solved thanks to the assistance as from your advice.
I will paste here the message that I received from the assistance. If someone has the same problem that is the solution.
--------------------------------------------------------------------------------------------------------------------------
If you want to retrieve a quarterly series of financial data, specifying the frequency will not be sufficient, because as you observed, fiscal year data will be returned.
The default period for financial data, unless specified otherwise, is Fiscal Year, so you need to make sure that you are requesting Fiscal Quarter information instead by adding Period=FQ0 i.e.
standard_option = {'Scale': 6, 'SDate': 0, 'EDate': -130, 'FRQ': 'Q', 'Curn': 'EUR','period':'FQ0'}
Another thing that I wanted to make you aware of, is that this particular company has a hybrid/mixed reporting pattern, which means that they report every 2nd quarter (March, September) and every 2nd Semi-Annual (not 4 quarters)
Using the fields for Revenue, Gross Profit and Net Income, you have now, you would not be able to get consecutive 4 quarters as they were simply not reported.
You would have to use equivalent fields that start with TR.F. (Instead of just TR. ex. TR.F.Totrevenue, TR.F.GrossProfIndPropTot, TR.F.NetIncAfterMinIntr), where this mixed reporting issue was mitigated by artificially deriving the quarterly data.0
Answers
-
As this forum is more for programming-type queries, rather than content queries - I would recommend you raise a ticket with our helpdesk via MyRefinitiv. That way a Content specialist can work closely with you and verify the assumption.
You can contact the Eikon Excel support team and ask for the fields and parameters which can be used with =TR function in Eikon Excel to get the quarterly data for revenue and other fields.
After that, you can use the same fields and parameters with the get_data method in Eikon Data API.
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 690 Datastream
- 1.4K DSS
- 629 Eikon COM
- 5.2K Eikon Data APIs
- 11 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 255 ETA
- 559 WebSocket API
- 39 FX Venues
- 15 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 25 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 279 Open PermID
- 45 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 23 RDMS
- 2K Refinitiv Data Platform
- 716 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 106 UPA
- 194 TREP Infrastructure
- 229 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 95 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛