About error: 'str' object has no attribute 'get'
Options
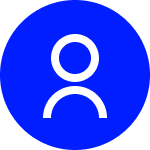
qi.chen
Newcomer
I'm using rdp to get data of private firms. I modified the code many times, but I kept get error like this. Could someone help me to fix this? Many thanks!
Below is my code:
import pandas as pd
import refinitiv.dataplatform as rdp
import time
import logging
def get_info(session, url, firm):
try:
endpoint = rdp.Endpoint(session=session,
url=url)
response = endpoint.send_request(
method=rdp.Endpoint.RequestMethod.GET,
path_parameters={"permId": firm},
)
logging.info(f"{firm} is processing!")
logging.info(f"Response is {response.status['http_status_code']}")
if response.is_success:
try:
wanted_response=response.data.raw['data']['businessClassification']['trbc']
logging.info(f"{firm} is completed!")
return pd.DataFrame([wanted_response],index=[f'{firm}'])
except AttributeError as e:
logging.error(f"error for {firm}: {e}!")
else:
logging.error(f"error for {firm}: {response.status['http_status_code']}!")
except Exception as e:
logging.error(f"An unexpected error occurred for {firm}: {e}")
return None
# Set configuration
session = rdp.open_platform_session(
"...",
rdp.GrantPassword(
username="...",
password="....."
)
)
url="https://api.refinitiv.com/user-framework/mobile/overview-service/v1/corp/business-classification/{permId}"
rdp.configure.config["http.request-timeout"] = 60
# import firm list
df_firm = pd.read_stata(...Data/Match/Match_data/final_company_sample.dta')
firm_list = df_firm['Company_PermID'].tolist()
filtered_list=[]
for value in firm_list:
if value != "":
filtered_list.append(value)
# get information of each firm
chunk_size=500
df=pd.DataFrame()
for i in range(0, len(filtered_list), chunk_size):
chunk = filtered_list[i:i + chunk_size]
for firm in chunk:
returned_df=get_info(session, url, firm)
if returned_df is not None:
df=pd.concat([df,returned_df],ignore_index=True)
logging.info(f"Completed processing chunk {i + 1} to {min(i + chunk_size, len(filtered_list))}")
time.sleep(1)
df.to_excel(".../Data/Industry_TRBC.xlsx",index=False)
Tagged:
0
Best Answer
-
Thank you for reaching out to us.
Refinitiv Data Platform Libraries are deprecated APIs.
Please upgrade the application to use the Refintiv Data Library for Python instead. The examples are on GitHub.
Then, you can enble logging in the RD library via the configuration file (refinitiv-data.config.json) to verify what the problem is.
{
...
"logs": {
"level": "debug",
"transports": {
"console": {
"enabled": false
},
"file": {
"enabled": true,
"name": "refinitiv-data-lib.log"
}
}
},
...Otherwise, you can enable logging in the API by using the following code.
import refinitiv.data as rd
rd.get_config()["http.request-timeout"] = 60
rd.get_config()["logs.level"] = "debug"
rd.get_config()["logs.transports.file.enabled"] = True
rd.get_config()["logs.transports.file.name"] = "refinitiv-data-lib.log"
rd.open_session()0
Answers
-
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 690 Datastream
- 1.5K DSS
- 629 Eikon COM
- 5.2K Eikon Data APIs
- 11 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 255 ETA
- 560 WebSocket API
- 39 FX Venues
- 15 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 25 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 281 Open PermID
- 46 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 23 RDMS
- 2K Refinitiv Data Platform
- 724 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 106 UPA
- 194 TREP Infrastructure
- 229 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 95 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛