ItemStream OpenAsync does not return a usable state or error code
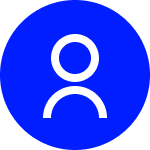
Similar to the 3.1.0 - Streaming - MarketPrice
Calling OpenAsync() always returns a stream state of "State.Closed". There is no way to tell if the stream will eventually be opened, or an error occurred and what the error was. When I look at the stream object in the debugger I can see some internal JSON string that would have the information but it is not accessible from the outside.
I would expect the stream state to be "Pending" if there was no error or add an additional property that indicates the error.
Best Answer
-
Hi @martin.grunwald,
When you issue the call:
var state = await stream.OpenAsync();
It will complete. That is, it will either be Opened or Closed. In your case, because you were not entitled to the data, it will be closed and never change after that. Once an item is closed, it will not go back to any other state. For example, if you request for an invalid item 'JUNK' for example or in your case for an item you are not allowed to see, it will not eventually open.
Within the Delivery layer, the Stream interface is a lower-level design-construct where the developer is required to use lambda expressions/callbacks to monitor behavior and status. The Stream interface is a long-lived interface where the status of the item can potentially change during its lifecycle. For example, it is entirely possible the backend service managing the item can be disrupted and thus a report will be sent out to the .OnStatus() callback reporting this fact. The best-practice to manage item requests is to use the .OnStatus() mechanism.
That being said, we have designed higher-level interfaces at the Content layer that provide simpler abstractions in case developers prefer not to use/understand how lambda/callbacks work. The StreamingPrices interface, outlined within examples 2.1.1 and 2.1.2 demonstrates how to use this interface. It is here that you can do the things I believe you were first trying. For example, you can query the status of an item you want to stream after the OpenAsync() returns. For example:
var item = "VOD.L";
... // Define request for item using StreamingPrices
var state = await stream.OpenAsync();
if (state != Stream.State.Opened)
{
Console.WriteLine($"Status for item {item}: {stream[item].Status}");
...
}The StreamingPrices interface provides the ability to request for multiple items within a single call. In addition, the StreamingPrices manages a cache so users of this interface can avoid using callbacks if they prefer. This of course depends on the complexity of what they want to achieve.
0
Answers
-
Can you advise what the StatusMsg reports? e.g. data state, text message etc - to indicate why the stream is closed?
e.g.
"State": {
"Stream": "Closed",
"Data": "Suspect",
"Code": "NotEntitled",
"Text": "Access Denied: User req to PE(122)"
}
The above indicates I am not permissioned for the item I requested.
Once a stream is closed, it will not be opened again unless you explicitly attempt to Open it again.
0 -
My point is: the necessary information is not available via the API.
In my test case I was not entitled to see the data, but this is besides the point. If my code calls OpenAsync() the API should tell me whether it worked or not. Using a debugger to manually hunt for some string is obviously not a solution.
0 -
I am not sure I fully understand your comment - however, I will pass your it onto the development team.
You are working with realtime streaming data - making an Async request to open a stream. If the API was able to submit the request to the server then it would deem the action to be a success - since it was able to submit the request to the server.
Once you make the OpenAsync() call the thread of control returns back to the application and continues executing any subsequent lines of code.
The server then responds asynchronously - at some time in the future with data on an open stream or with closed stream e.g. because you are not permissioned or the RIC does not exist - then this would be reported asynchronously in the status msg.
Therefore, you should be processing the StatusMsg - rather than using the debugger.
If however, you want to know the state of the stream before proceeding then you should use the regular Open() call. You will still need to interrogate the StatusMSg to identify the reason for the Closed stream.
0 -
I have also shared your concern with the Dev team - so hopefully they may respond as well - in case I misunderstood your concern
0 -
I think I noticed my mistake. After switching to OpenAsync I was expecting the returned state to be valid instead of waiting for the callbacks. I am currently testing this.
0 -
Hi @martin.grunwald,
The OpenAsync() call is typically designed to work with the await keyword. When you call OpenAsync() it will return a task. If you want to get the actual return value from the Task, you have to await it. Perhaps you can describe what you want to do.
For example, looking at the 3.1.0 MarketPrice example, if I change it to look like this:
The OpenAsync() call above returns right away. The variable 'state' is a Task that you must await. If you want to get the actual state of the stream immediately after you make the call, the stream interface provides a convenient property call 'OpenState', which you can see above.
When I run this code above, you will see the open state right after the call is 'Pending' then the data eventually comes in via the supplied callbacks, i.e.
In your specific case, if the data is invalid or you are not permissioned, you will still see a pending right after the call. Once the call is fulfilled, i.e. a response comes back, the state will changed to 'Closed' (in your case). If the request is valid, the state would be 'Opened'.
0 -
My original implementation used:
var state = await stream.OpenAsync();
My observation was that the "state" variable is always "closed" whether the call will eventually succeed or not.
I solved my issue by ignoring the "state" altogether and relying on the "OnStatus" callback to deliver the error state.
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 690 Datastream
- 1.4K DSS
- 629 Eikon COM
- 5.2K Eikon Data APIs
- 11 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 255 ETA
- 559 WebSocket API
- 39 FX Venues
- 15 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 25 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 280 Open PermID
- 45 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 23 RDMS
- 2K Refinitiv Data Platform
- 721 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 106 UPA
- 194 TREP Infrastructure
- 229 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 95 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛