Eikon messenger API
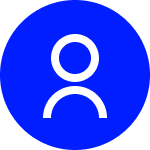
I am currently working on a project that requires extracting discussions from different group chats in Eikon Messenger using Python. To proceed, I need the following information and credentials:
Eikon Data API AppKey
Messenger Bot API credentials (bot_username, bot_password, messenger_appkey)
Additionally, I would appreciate it if you could provide the documentation or any guidelines on how to set up and use these APIs to extract the discussions.
Could you please help on this project?
Thank you for your support.
Best regards,
Ben
import eikon as ek import requests import websocket import json import threading # Initialize Eikon Data API ek.set_app_key('YOUR_EIKON_APPKEY') # Messenger Bot API credentials bot_username = 'your_bot_username' bot_password = 'your_bot_password' messenger_appkey = 'your_messenger_appkey' messenger_ws_endpoint = 'wss://api.collab.refinitiv.com/services/nt/api/messenger/v1/stream' # Authentication function def authenticate(): url = 'https://api.refinitiv.com/auth/oauth2/v1/token' headers = { 'Content-Type': 'application/x-www-form-urlencoded' } data = { 'grant_type': 'client_credentials', 'client_id': messenger_appkey, 'client_secret': bot_password } response = requests.post(url, headers=headers, data=data) return response.json()['access_token'] # WebSocket function to receive messages def on_message(ws, message): print(f"Received message: {message}") def on_error(ws, error): print(f"Error: {error}") def on_close(ws, close_status_code, close_msg): print("### closed ###") def on_open(ws): print("### opened ###") # Main function to start the WebSocket connection def start_websocket(): ws = websocket.WebSocketApp(messenger_ws_endpoint, on_open=on_open, on_message=on_message, on_error=on_error, on_close=on_close) ws.run_forever() if __name__ == "__main__": # Authenticate and get the access token access_token = authenticate() # Start the WebSocket connection to receive messages websocket_thread = threading.Thread(target=start_websocket) websocket_thread.start()
Answers
-
Hello @Ben
Please be informed that the Messenger Bot API is a feature completed API (legacy). There is the new Messenger User Feed for LSEG Messenger that will replacement to it. It is expected to be launched in Q2 2025.
If you cannot wait, please contact your Account Manager or LSEG representative to help you with the Messenger Bot account.
About the app key, you can use the App-Key Generator on the Workspace desktop application.
Once you are in the App-Key Generator app, input your app name and check the "EDP API" box, and then click register new app.
Please note that the Eikon Data API is not related to the Messenger Bot API. The following code is not needed:
import eikon as ek # Initialize Eikon Data API ek.set_app_key('YOUR_EIKON_APPKEY')
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 685 Datastream
- 1.4K DSS
- 615 Eikon COM
- 5.2K Eikon Data APIs
- 10 Electronic Trading
- Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 252 ETA
- 556 WebSocket API
- 38 FX Venues
- 14 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 23 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 275 Open PermID
- 44 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 22 RDMS
- 1.9K Refinitiv Data Platform
- 652 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 104 UPA
- 193 TREP Infrastructure
- 228 TRKD
- 917 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 90 Workspace SDK
- 11 Element Framework
- 5 Grid
- 18 World-Check Data File
- 1 Yield Book Analytics
- 46 中文论坛