How to handle COMPLETION_EVENT while migrating Refinitiv RFA API to EMA API?
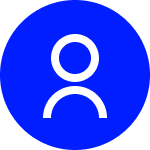
We are currently upgrading our application from RFA API to use EMA API. We referred a similar question in this link:
https://community.developers.refinitiv.com/questions/92298/whats-the-equivalent-of-rfas-completion-event-in-r.html
But we are not able to use the rsslIsFinalState given in the solution here. Please provide a working example to identify the completion event or closing of a stream in any Consumer(given below) implementing OmmConsumerClient.
///*|----------------------------------------------------------------------------------------------------
// *| This source code is provided under the Apache 2.0 license --
// *| and is provided AS IS with no warranty or guarantee of fit for purpose. --
// *| See the project's LICENSE.md for details. --
// *| Copyright (C) 2019 Refinitiv. All rights reserved. --
///*|----------------------------------------------------------------------------------------------------
package com.refinitiv.ema.examples.training.consumer.series100.ex100_MP_Streaming;
import com.refinitiv.ema.access.Msg;
import com.refinitiv.ema.access.AckMsg;
import com.refinitiv.ema.access.GenericMsg;
import com.refinitiv.ema.access.RefreshMsg;
import com.refinitiv.ema.access.ReqMsg;
import com.refinitiv.ema.access.StatusMsg;
import com.refinitiv.ema.access.UpdateMsg;
import com.refinitiv.ema.access.EmaFactory;
import com.refinitiv.ema.access.OmmConsumer;
import com.refinitiv.ema.access.OmmConsumerClient;
import com.refinitiv.ema.access.OmmConsumerConfig;
import com.refinitiv.ema.access.OmmConsumerEvent;
import com.refinitiv.ema.access.OmmException;
class AppClient implements OmmConsumerClient
{
public void onRefreshMsg(RefreshMsg refreshMsg, OmmConsumerEvent event)
{
System.out.println(refreshMsg);
}
public void onUpdateMsg(UpdateMsg updateMsg, OmmConsumerEvent event)
{
System.out.println(updateMsg);
}
public void onStatusMsg(StatusMsg statusMsg, OmmConsumerEvent event)
{
System.out.println(statusMsg);
}
public void onGenericMsg(GenericMsg genericMsg, OmmConsumerEvent consumerEvent){}
public void onAckMsg(AckMsg ackMsg, OmmConsumerEvent consumerEvent){}
public void onAllMsg(Msg msg, OmmConsumerEvent consumerEvent){}
}
public class Consumer
{
public static void main(String[] args)
{
OmmConsumer consumer = null;
try
{
AppClient appClient = new AppClient();
OmmConsumerConfig config = EmaFactory.createOmmConsumerConfig();
consumer = EmaFactory.createOmmConsumer(config.host("localhost:14002").username("user"));
ReqMsg reqMsg = EmaFactory.createReqMsg();
consumer.registerClient(reqMsg.serviceName("DIRECT_FEED").name("IBM.N"), appClient);
Thread.sleep(60000); // API calls onRefreshMsg(), onUpdateMsg() and onStatusMsg()
}
catch (InterruptedException | OmmException excp)
{
System.out.println(excp.getMessage());
}
finally
{
if (consumer != null) consumer.uninitialize();
}
}
}
Best Answer
-
Thank you for reaching out to us.
The code looks like this:
public void onRefreshMsg(RefreshMsg refreshMsg, OmmConsumerEvent event)
{
...
if (isFinalState(refreshMsg.state())) {
...
}
...
}
public void onStatusMsg(StatusMsg statusMsg, OmmConsumerEvent event)
{
...
if (statusMsg.hasState()) {
System.out.println("Item State: " +statusMsg.state());
if (isFinalState(statusMsg.state())) {
...
}
}
...
}
public boolean isFinalState(OmmState state) {
if(state.streamState() == StreamState.CLOSED ||
state.streamState() == StreamState.CLOSED_RECOVER ||
state.streamState() == StreamState.CLOSED_REDIRECTED ||
state.streamState() == StreamState.NON_STREAMING) {
return true;
}
return false;
}The state is available in the Refresh messages and optional in Update messages.
You need to check the stream state. If the stream state is CLOSED. CLOSED_RECOVER, REDIRECTED, or NON_STREAMING, this means that the stream has been closed.
0
Answers
-
Please provide a running example in Java
0 -
Thanks for the prompt reply
0
Categories
- All Categories
- 3 Polls
- 6 AHS
- 36 Alpha
- 166 App Studio
- 6 Block Chain
- 4 Bot Platform
- 18 Connected Risk APIs
- 47 Data Fusion
- 34 Data Model Discovery
- 689 Datastream
- 1.4K DSS
- 627 Eikon COM
- 5.2K Eikon Data APIs
- 11 Electronic Trading
- 1 Generic FIX
- 7 Local Bank Node API
- 3 Trading API
- 2.9K Elektron
- 1.4K EMA
- 255 ETA
- 559 WebSocket API
- 39 FX Venues
- 15 FX Market Data
- 1 FX Post Trade
- 1 FX Trading - Matching
- 12 FX Trading – RFQ Maker
- 5 Intelligent Tagging
- 2 Legal One
- 24 Messenger Bot
- 3 Messenger Side by Side
- 9 ONESOURCE
- 7 Indirect Tax
- 60 Open Calais
- 279 Open PermID
- 45 Entity Search
- 2 Org ID
- 1 PAM
- PAM - Logging
- 6 Product Insight
- Project Tracking
- ProView
- ProView Internal
- 23 RDMS
- 2K Refinitiv Data Platform
- 708 Refinitiv Data Platform Libraries
- 4 LSEG Due Diligence
- LSEG Due Diligence Portal API
- 4 Refinitiv Due Dilligence Centre
- Rose's Space
- 1.2K Screening
- 18 Qual-ID API
- 13 Screening Deployed
- 23 Screening Online
- 12 World-Check Customer Risk Screener
- 1K World-Check One
- 46 World-Check One Zero Footprint
- 45 Side by Side Integration API
- 2 Test Space
- 3 Thomson One Smart
- 10 TR Knowledge Graph
- 151 Transactions
- 143 REDI API
- 1.8K TREP APIs
- 4 CAT
- 27 DACS Station
- 121 Open DACS
- 1.1K RFA
- 106 UPA
- 194 TREP Infrastructure
- 229 TRKD
- 918 TRTH
- 5 Velocity Analytics
- 9 Wealth Management Web Services
- 96 Workspace SDK
- 11 Element Framework
- 5 Grid
- 19 World-Check Data File
- 1 Yield Book Analytics
- 48 中文论坛